Typescript Tuples are special types that is used to define types for each element in an array. There are different data-types available in typescript for performing high complex operations including, string, number, interface, any, Boolean, object, arrays and interface. You can use arrays as stack to define list of items of the same types, but what if you want each element in the list to have a different type? It will be somehow challenging, although you can define an interface which have different types of properties and pass it as a generic type to your array. This will work but you will have to write a lot of code, however you can easily get this done in a single line of code using tuples. But before I show you, let me explain…
What is tuple in typescript?
Table of Contents
A typescript tuple is a pre-defined array of fixed length and types for each element. They are just arrays, however the index element in such array has their own types already defined during the initiation of the tuple. When a tuple is declared without the readonly keyword, you can add or push new elements to it. The data-type of the new element will be determine based on its value. For example, if you push “JavaScript”, the type will be a string but if there’s no string type defined in the tuple, it will throw and exception.
The problem tuples solve is that, it helps to specify individual types for each element in an array. This is not possible with regular array and that’s the reason why tuple was introduced.
Let me show you some examples and how easily it is…
Tuple example in typescript
To help you understand how to apply tuples, consider the below example of how it different from other types like number, string, boolean and interface.
Tuple example vs other data-types…
var studentId: number = 5;
var studentName: string = "Justice";
// Tuple type variable
var student: [number, string] = [5, "Justice"];
You can see from the above example, I have declared two variables studentId
of type number and studentName
of type string with pre-defined values. But instead of two separate variables, we can achieve the same thing with tuple type in a single-line of code. Have a look at the above last-line of code and will see how tuples makes life easy. Tuples of this type is common in react if you have noticed.
Check also: JavaScript VS Python: Real-World Application Difference
How do you access tuple elements in TypeScript?
Elements in tuple can be accessed using their index just as you would in a regular array. Tuple is really easy to work with and you can use it to access all the methods and properties an array has such as length and push()
.
Example: accessing tuple variable type…
// Tuple type variable
var student: [number, string] = [5, "Justice"];
student[0]; // return 5
student[1] // return Justice
Tuple elements index begins from zero and it increased by default to the last index. When a new element is added or removed, it will adjust it element index in order just like a regular array. The above example illustrate how you can access each element in a tuple by it index.
Check also: How to validate an email address in JavaScript
Add Elements to Tuple
If you have a tuple already declared with or without element, you can add new element to the tuple by using the push() array method. This method will push element to the end of the tuple, if you want to inserts new elements at the start of an tuple use the unshift()
method.
Example using the push() method to add element at the end of a tuple…
// tuple variable
var student: [number, string] = [5, "Justice"];
student.push(6, "James");
console.log(student); //Output: [5, "Justice", 6, "James"]
Example using the unshift()
method to add element at the start of a tuple…
// tuple variable
var student: [number, string] = [5, "Justice"];
student.unshift(6, "James");
console.log(student); //Output: [6, "James", 5, "Justice"]
Because the tuple is defined with a type of number and a string, the above example does work successfully. But what if we try to insert Boolean type value? It will throw an exception. Let me show you…
var student: [number, string] = [5, "Justice"];
// Error: Argument of type 'boolean' is not assignable to parameter of type 'string | number'
student.push(6, false);
console.log(student);
The above code couldn’t be compiled to the console.log(student)
line-of-code because there is an error on the line before it saying “Argument of type ‘boolean’ is not assignable to parameter of type string | number”. Boolean value is not permitted because a tuple of type string and number can only store values of such types.
Check Also: How to install Angular 10 CLI
Readonly Tuple
The best way to define a tuple is to prevent it from being modified. And you can do that by adding the “readonly” keyword to your tuple type. Because the initial values of tuples elements have a strongly defined types with fixed length.
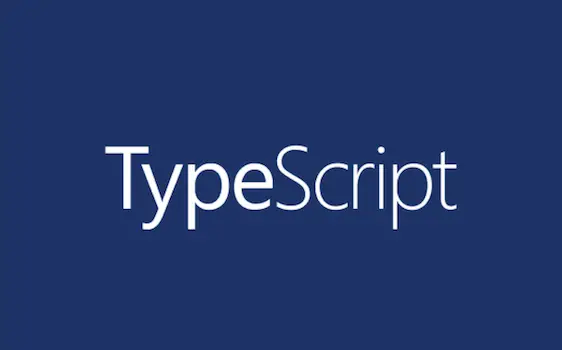
Example of read only tuple
// Create a tuple of type readonly. And provide tyes for each of it value
// Meaning it values must be in order of types: string, boolearn, number
let personDetials: readonly [String, boolean, number];
// initialize the tuple values
personDetials = ["justice", true, 55];
/*
You can also write the above tuple on a single line as
const personDetials: readonly [String, boolean, number] = personDetials =["justice", true, 55];
*/
console.log(personDetials); // Results: [ "justice", true, 55 ]
// Error: Property 'push' does not exist on type 'readonly [String, boolean, number]'
// why becuase, you can't modify the value of readonly var, you can only fetch/read it value
personDetials.push("justice");
When you try to insert a value to a tuple of readyonly type, you will get an error saying “Property ‘push’ does not exist on type ‘readonly [String, boolean, number]”. That’s because it length is fixed and you can’t modify it by appending new ones into it.
Check also: How to make money coding for beginners.
Typescript tuple array
Typescript tuple array can be used to define multi-dimensional array known as an array within an array. You have to be careful using it especially if you don’t have strong knowledge of looping through regular arrays.
How to declare tuple array and iterate it elements using map()
method.
// Define a tuple that will take an array
let myTuple: [String, number][];
// pass different values of array to the tuple
myTuple = [["justice", 21],["Shadrack", 39]];
// Loop through the tuple
myTuple.map((eachTuple)=>{
// Grab each first element in each tuple
console.log(eachTuple[0]);
});
/*
result:
Justice
Shadarck
*/
Typescript – named tuple
Named tuples gives us the opportunity to provide names or context for each element in the tuple. So, you can give a name for your tuple fields when defining it. This helps you to know the structure of it. However, it important to know that you can’t access the tuple values with their name, you can only do so with their index.
Named tuple example
// Define a tuple with a field name and it data-type
let person: [firstName:String, age:number];
// initialize the
person= ["justice", 21];
// access the values with index not it name
console.log(person[0]);
/*
result:
Justice
*/
Typescript typed tuple
You can use tuples as type for your variables just as you would with other types such as string and numbers. And you can declare such variables with the const or the let keyword together with access modifiers like private, protected and public.
Check also, how to think like a programmer.
Typed tuple example…
// define a tuple
let employee: [number, string]=[5, "justice"];
// assign the above tuple
// this will let newEmployee have a type of [number, string] which is the same as the tuple above
let newEmployee=employee;
console.log(newEmployee);
// Output: [ 5, "justice" ]
Typescript return tuple example
typescript tuple can be used as a return type or and argument of a function. Although you can’t specify a return type of “tuple” in your method, what you can do is to just return a tuple in the method.
Example of a method that takes tuple argument and returns it…
// Create a tuple
const somePerson:[String, number] = ["justice", 21];
// call the below method and pass in the above tuple as argument
// But make sure your tuple has the same order & number of types as your function argument
this.printPerson(somePerson);
// Create a function that takes a tuple parameter
printPerson(person: [firstName: String, age:number]){
// let the method return the tuple
return personalbar;
}
// Output: ["justice", 21];
When should I use tuple TypeScript?
You should use tuple when you want to create an array of element having different types. That’s when a tuple should be used, if you’re going to define a list of items that will have the same data-type, simple use regular array.
You have learnt a lot in this lesson, if you’re a angular, react or vue fronted developer, this will help you a lot on your journey.
Check also how you can improve your typescript skill by learning angular for web development.