Learn how to validate an email address in JavaScript with this simple method. If you are a web developer, you surely know why you should validate all the requests that will be sent by the client.
And yes, what I mean by that in simple terms is “Don’t trust any input request sent by the client.” Most clients are hackers themselves and to protect your application, you surely need to verify all the client input to see if it contains the right credentials that you need.
So how can I perform email validation in JavaScript?
If you are a pro in web development or a beginner, you don’t need to worry at all. Because am going to give you a simple hack that will help you to perform validation with a few lines of code.
But before I show you the hack, I first want you to know…
What is Email Validation and Why You Should learn it?
Table of Contents
So in a real-world application, the user will surely send some credentials to the server. Please note that, am going to be referring to “client” as a “user” of an application. So when you hear me mentioning any of it, am actually referring to the person who is performing some action with the application or the web-app.
So what happens is that, as a developer you have to verify all the input request that the client will send to the server.
Hey, that’s it mean I can only perform validation on the server?
No, you can very users’ credentials on the client before being sent to the server for further process. And likewise, you can also validate the user’s credentials on the server as well before being sent to the database.
Check also: Java Vs JavaScript: Which Is Better
So email-validation is simply the act of verifying client emails to see if it’s correct or wrong, simple.
Although clients know their emails very well as I said, you don’t have to trust all their input requests. Because, what if a client made a typing error when trying to sign-up.? Meaning he won’t be able to login to his account when he tries to with his correct email address.
Even though this may not have any effect on you the programmer. But think about it…
What if the credentials sent contains some script to hack your database?
What if the client is actually trying to hack already registered users details?
So you can surely see that validating users’ credentials is really a must and not something you should skip over.
But how can you validate emails without knowing how emails look like?
Structure of an Email Address
An email is a list of string characters that is divided into two-part with the @ symbol. So is just separated into left and right sections. The left part represents the user-personal-info and the right part represents the domain of the email.
The personal-info may have a length up to 64 characters and the email domain could be up to 254 characters long. And that’s the exact length every email address can’t go beyond.
So here is an example of an email – justice@tutorialscamp.com.
From the above example, you can see that I have my personal-info on the left and the email-domain on the right.
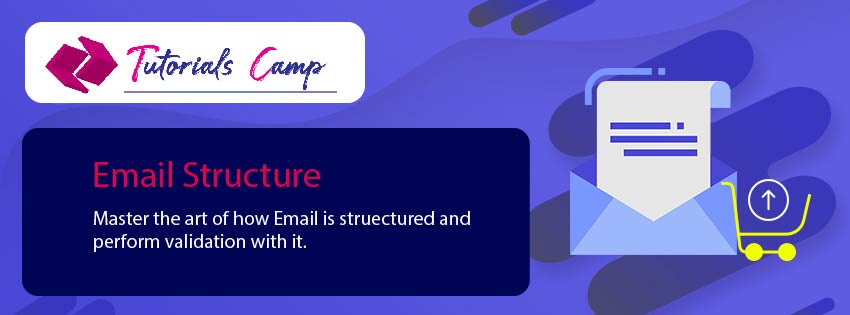
A typical email address personal-info should be of these ASCII symbols or characters:
- Uppercase characters (A-Z) lowercase Characters (a-z)
- It can contain numbers of (0-9)
- Special symbols of [$#! ~{|}’_^?=-+*&% ]
The email-domain could be of .com, .info, .net depending on the server that is hosting it. And these characters can contain hyphens, dots and digits or numbers.
Example of Valid Email Address
Example of invalid email Address
- Peter.tutorialscamp.com (This is wrong because there is no @ symbol)
- @tutorialscamp.com (Wrong because there is no personal-info before the @)
- tutuorialscamp@facebook.b (Not valid because of “.b”)
- tutorialscamp…justice@youtube.com (Wrong because double dots are not allowed in emails)
- .tutorials@gmail.com (can’t start an email with “.”)
So all these are examples of hacks you should perform email validation against. Never allow any of these emails to be passed in your database because such emails are considered to be invalid.
But if you don’t know how to do it, don’t worry I have got you covered with an easy method below…
Different Methods to validate Email in JavaScript
There are so many ways you can validate email addresses in JavaScript. And you don’t have to worry because am going to walk you through with an easy way of doing it. But mostly, below are the popular methods to perform email validation:
- Using the regex or regular expression (my preferred way)
- Using plain HTML built-in email validation (Very easy)
- Using other third party libraries (recommended but there are pros and cons to it)
Those are the best ways most top programmers prefer that’s the “email validation regex JavaScript method.” Plain HTML can also do it but you need to be very careful in case you want to use a third-party library.
Check also: JavaScript vs python: which is better?
Why because these libraries provide a CDN which most programmers use it to get access to their functions. These libraries are being hosted on an external server, when the server goes down, anyone can register in your application without undergoing any email validation. (Be-careful my friend, not all sources are trustworthy)
Maybe you didn’t know, but using regex expression is the most preferred approach. Remember, you are getting to the important part of how to validate an email address in JavaScript?
Let me show you how…
How to Validate Email Address with Regex Expression in JavaScript
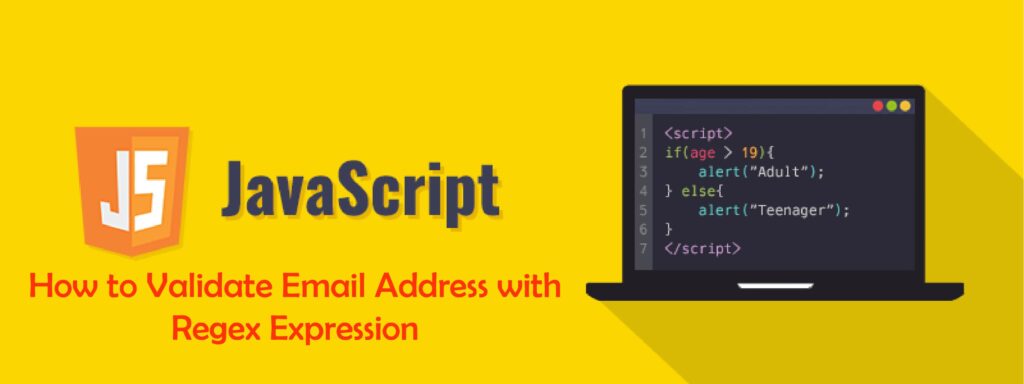
When it comes to email validation with regular expression, there is no general method. But we rather use a pattern, and every developer out there is using his or her own set of regex pattern for validation.
So what is the proven pattern?
I knew you will surely ask such question, but what if I tell you it already solved?
Don’t worry am going to give you the proven pattern used by top developers for performing email-validation. I will also walk you through how to go about it, so there is nothing to be bordered about because is really easy.
Here is the pattern that is proven by top developers to validate (99.8%) of all emails:
/^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/
But the question is “how can you memories and use this guaranteed regular expression pattern?”
So without any nonsense code, let go straight to the point of…
Regex Example using JavaScript to Validate Email Addresses
First, you don’t need to memorize this long pattern, All you need to do is save it somewhere and let me show you how easily you can use it to validate any email. If you think saving this pattern is a challenge, then just bookmark this guide URL and simply click on it whenever you need it.
First, let create an HTML element with just one button. Am actually going to use inline-JavaScript but you can go ahead and save your script to a separate .js file and just provide the link in the HTML file.
Check also: How to install angular 10 CLI
So in all here is how my code looks like, explanation is provided below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<!-- CLICK BUTON THAT CONTAINS USER EMAIL -->
<button onclick="validatEmail('java@gmail.B')">Validate Email</button>
<!-- SCRIPT TO VALIDATE THE USER EMAIL -->
<script>
// CREATE A FUNCTION WITH PARAMETER
function validatEmail(email){
//ASING THE REGEX EMAIL VALIDATION PARTERN TO A CONSTANT
const pattern = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
// CHECK IF THE USER-EMAIL FOLLOWS THE ABOVE REGEX-EMAIL-VALIDATION-PARTTERN
if(pattern.test(email.toLowerCase())){
// if is correct print out "valid email"
console.log("valid email");
}
else{
//else print "invalid email"
console.log("invalid email");
}
}
</script>
</body>
</html>
What is going on in the above script?
Well it just in these few steps:
- I have defined a function with a parameter (remember the parameter is the email the user will pass, in our case, it passed in the HTML on-click button which is calling the function)
- Then inside the function, I have assigned the regex-email-pattern to a constant variable “pattern”
- Then I check if the user email is valid with the “test()” method after it’s converted into lowercase. (Remember, the test() method performs a such for a match between a string and a regular expression. It returns a Boolean of Yes or No)
- If the email is correct, print out “valid email”
- If the user email is no correct, print out “Invalid email”
It really easy to understand so let test it in the browser.
Testing the above Example
First, let me pass in the wrong email of “..java@gmail.com” and you will see an “invalid email” below in the console because earlier on I told you that, double dots are not allowed. So look at the console below for the error.
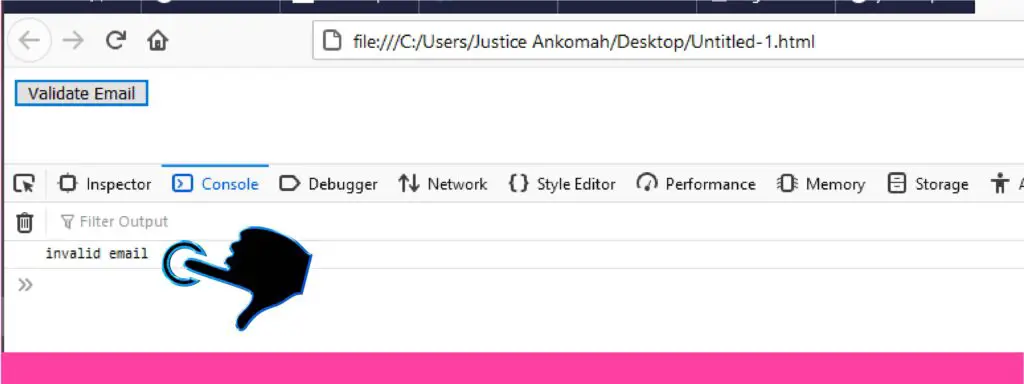
And let me pass in a correct email of “java@gmail.com” and you can see its printed “valid email” in the console.
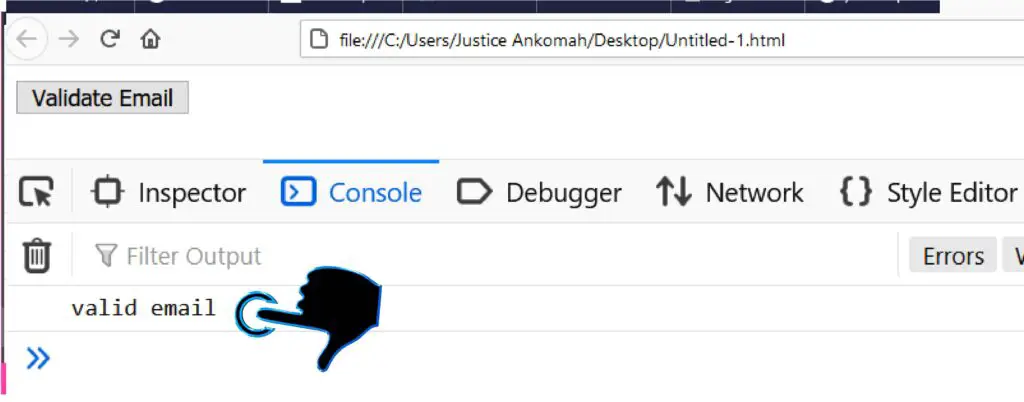
That’s how easily you can do JavaScript Email validation using Regex Expression. If you have any difficulty at this point, let me know in the comment section below.
Check also: Angular 10 introduction for beginners
HTML5 Email Validation
If you want a simple way to validate a user’s credentials, html5 comes with an easy way to do this with a single line of code. You don’t need to do anything hard with this approach, because is really easy and all you have to do is to make use of the html5 “email input attribute.“
Don’t be worried am going to show you how to do it.
Html5 input type=”email” Validation Example
All you need is a simple login form with a submit button. Because here the user needs to perform some action on the client before you can check the validity of his or her input. So simply create an HTML file with the below code. But take notice of the type input=”email” don’t be worried about the method that is behind it because is taken care of by html5 developers
<form>
<input type="email" placeholder="Enter your email">
<input type="submit" value="Submit">
</form>
Now if I test this out with a wrong email “….java@gmail..com” you can see the error below…
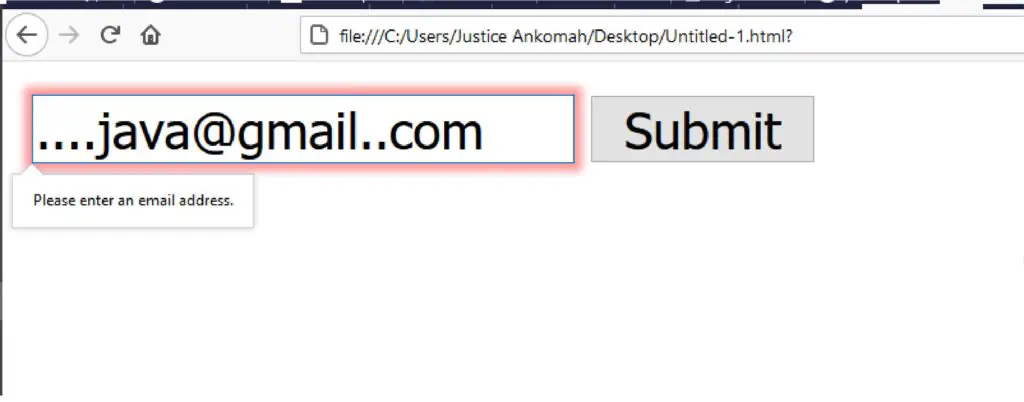
Check also: How to use illustrator shape builder tool
Which Method should You Use?
You can go ahead and use regex email validation in JavaScript Or plain html5. But mostly it depends on the level of security you want your application to have.
But what top programmers do is just use both of the above two methods together. If you think that’s not possible, then it is. You just have to pass the user email to the script function that will perform the validation. Very simple, because I see most of the top programmers doing the same and it’s my preferred approach too. But let me warned you again, don’t use third-party libraries. (I have been a victim once and it cost me a lot, so save yourself, my friend.)
Conclusion: simple email validation in JavaScript
That is all to it, my friend, if you are a front-end or back-end developer, don’t underestimate the act of checking users’ credentials before passing it to the server. Because hacking is real and other software developers have a bot that they sometimes use to attack other sites.
Check also: JavaScript vs jQuery key difference
So be security-minded in your programming journey and keep hackers out of your way. That’s How to validate an email address in JavaScript with regular expression and html5.
If you think this guide is so helpful or you face some challenges, please let me know in the comment box below. Also, take a look at how this next guide will help you to become a good programmer.
This post is priceless. When can I find out more?