When you create a new Java program and you initialize a variable or method outside the main method, and you try to execute or reference it, you will encounter a compile time error “Cannot make a static reference to the non-static method or a non-static field”. It doesn’t matter what programming language you use either c#, JavaScript, python, C++ or Java, you will encounter such error unknowingly.
Both well experienced and newbie programmers make this mistake unknowingly of accessing non-static methods or fields from a static context.
Example Error of “Cannot make a static reference to the non-static method or a non-static field” in Java.
package myjavaapp;
public class Main {
String firstName = null;
public static void main(String args[]) {
System.out.println("In main method");
firstName = "Justice";
displayFistName();
}
void displayFistName(){
System.out.println("Your First Name is: " + firstName);
}
}
When we run the above application, this is the error we will get below reference error…
Exception in thread "main" java.lang.Error: Unresolved compilation problems:
Cannot make a static reference to the non-static field firstName
Cannot make a static reference to the non-static method displayFistName() from the type Main
at myjavaapp.Main.main(Main.java:16)
The above exception is a compile-time error not a run-time error. You can see that the java compiler cannot compile the above code. Why because, if you take a clear look at the source-code above, you can see that the main method is define with a “static” keyword. Which simply means, the Java virtual machine (JVM) should execute it without creating an object of the class. Well if that is the case, we have the “firstName” field and “displayfistName()” which are non-static inside the static main “method”. The compiler will definitely complain because the context of the object reference isn’t the same.
Check the image below to see how the source-code looks like. I want you to mainly focus on where the class properties and methods are being defined and accessed.
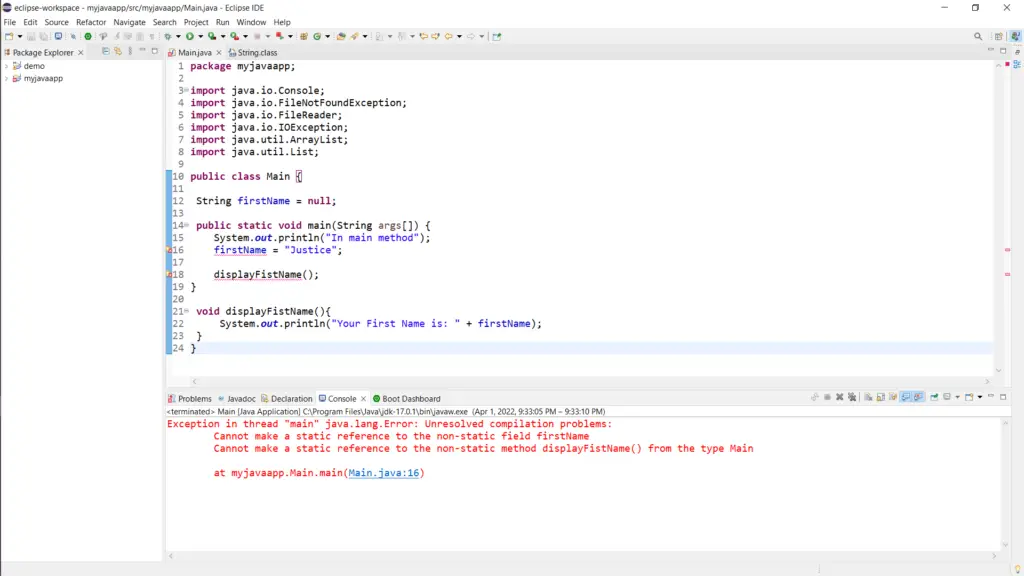
In every Java class, there is static fields and methods, instance and local variables. And you have to understand the logic of each field or method and how it relates to the class and it objects. Let me show you the difference and the after I will teach you how to fix the error of accessing non static fields and methods in a class.
- Class Static fields/variables and methods – Static variables are declared with the static keyword with access modifies like private, public and protected. They belong to the class and not the class instance and does because they are accessed direct without creating object of the class. You can choose to declare such fields as properties of a class or static method. You can’t define static variable in a non-static method. Throughout the operation with the class, you will have only one copy of static field, this helps to improve performance because it saves memory.
- Instance variables – Instant fields or variables actually represent the class itself whenever you implement a singleton-pattern. Such variables can be both static and non-static depending on the condition where it might be needed. Its initiation takes places when an object of the class is created, which means inside the class, its accessible in methods, class block and the constructor.
- Local variables – The opposite of global fields is local variables which is defined inside a particular block of scope. For example, if you declare a local field inside a specific method, such variable can be accessed only inside the method block. But in JavaScript things work different, you can declare and initialize a variable inside a constructor and access it in different methods.
Check also, Java Vs JavaScript Programming Language (Which Is Better?)
Solutions: static reference to non-static fields/variables
Table of Contents
How to access static fields/methods in different blocks is what am about to show you.
1. Static variables can be accessed or reference using the class name
Since static fields belong to the class itself and not its instances, you can use the class name to access those variables.
public class Main {
public static void main(String args[]) {
// Access the static field directly using the class Name
System.out.println(Person.firstName);
}
}
class Person{
// Declare a static field
static String firstName = "justice";
}
Inside the above code, you can see that without creating an object of the Person class am able to access the static “firstName” property.
2. Static Fields Can be referenced in a static block
Since like attract like, You can access static variables in similar scopes like static methods.
package myjavaapp;
public class Main {
// Declare a static field
static String firstName = "justice";
public static void main(String args[]) {
// Access the static field directly without using "this" keyword
System.out.println(firstName);
}
}
How to reference non static fields/methods?
You can create an object of a class to access it static fields and methods both outside and inside of the class. Let try and achieve this by taking the first exception we started with “Fix cannot make a static reference to the non-static method or a non-static field” in Java.
package myjavaapp;
public class Main {
String firstName = null;
public static void main(String args[]) {
// Create an object of the class
Main main_obj = new Main();
main_obj.firstName = "Justice";
main_obj.displayFistName();
}
void displayFistName(){
System.out.println("Your First Name is: " + firstName);
}
}
// Output:
// Your First Name is: Justice
You can see from the above that by creating an object of the “Main” class were able to access it non static fields in the main method. Mostly you would do the same when you want to reference it outside the scope of the class. See the below image for the complete source code.
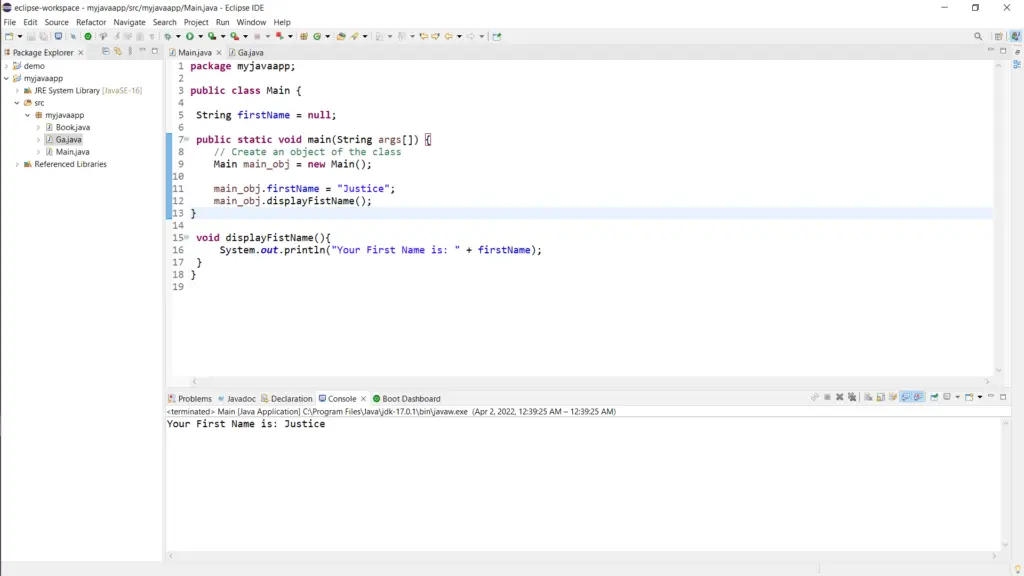
How do you assign a non static variable to a static variable?
You can not directly assign a non-static variable to a static variable because they don’t have the same context and such reference won’t match. However since we know static properties can be accessed directly without creating an object of the class, we can do so with ease. What you can do is to create an object of the class and use it to access it non static variables. And you can use such reference to assign the variable to static fields or use the variable in static methods.
Java example: Assigning Non Static Variable to Static Variable
public class Main {
// create a static variable
static String staticVariable = "Static Variable";
//create none static variable
String nonStaticVariable = "Non Static Variable";
public static void main(String args[]) {
// Create an object of the class
Main main_obj = new Main();
// Assign the non-static variable to the static variable
Main.staticVariable = main_obj.nonStaticVariable;
// print the static variable
System.out.println(Main.staticVariable);
// output:
//Non Static Variable
}
}
Kindly look at the source-code compiled in eclipse IDE below together with the output.
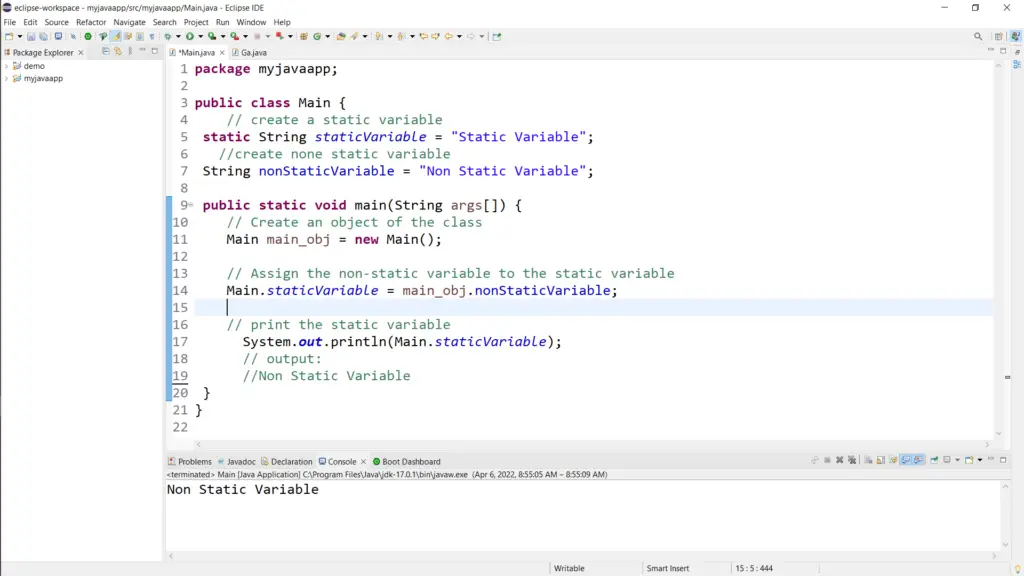
Read the Step-by-step guide here: 8 Proven Ways To Learn How To Think Like A Programmer: For (Creative Problem Solving)