Learn how to run JavaScript files in the terminal or from the command prompt by using the NodeJs runtime environment. JavaScript is a scripting language for making web pages interactive that means it’s interpreted in the browser only. Therefore you can only run js file in the terminal if only you have NodeJs installed on your computer.
So we first need to install it, so open this link to download NodeJs runtime environment. Then open the downloaded .exe file and install it on your system globally. If you have it installed on your system already, then you are free to skip this step.
We are good to run js code in the terminal now so let get started.
Example 1 – Running a JS Script from the Command Line
Table of Contents
First, create a new JavaScript file and name it “helloworld.js”. You can simply use any text editor you have or if you have visual studio code installed then use that. The key here is to make sure that you name the file with .js extension.
Now, let create a basic JS script that output the name and age of someone using a function. You can copy and paste the below code into the file you have created to save time.
// create a function
function outputNameAndAge(name, age) {
return "My name and age is: " + name + " " + age;
}
// execute the function
console.log(outputNameAndAge("Justice", 20))
When you run the above code directly in the browser the output will be, My name and age is Justice 20 Check also, Java Vs JavaScript: Which Is Better?
Steps to run js code in the terminal
- Open your command prompt or the terminal by typing cmd on your system search box. You can choose to open it as an administrator or not.
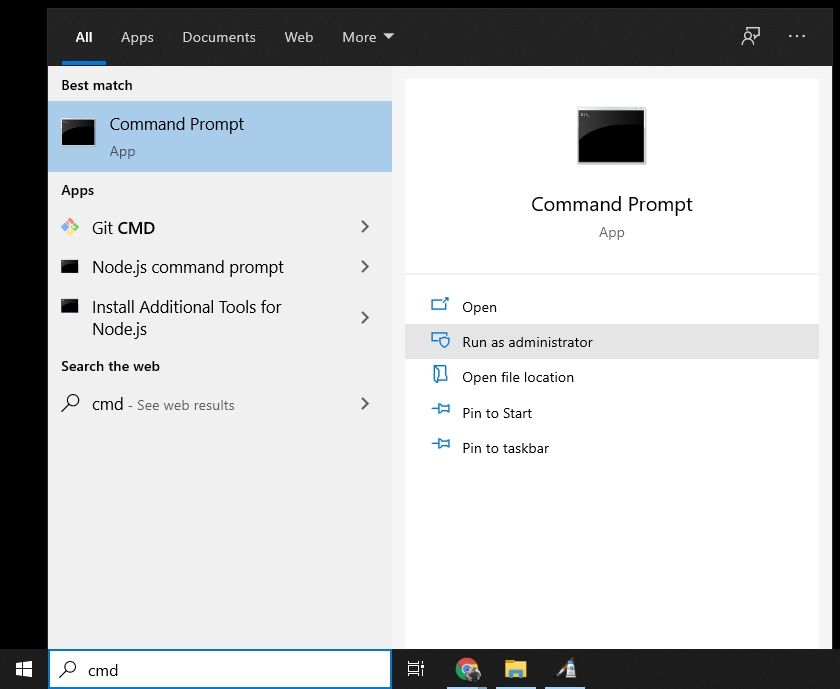
- Use cd to navigate to the path where you saved the above file. If you ignore this step it will not work because your system can’t find the path of the js file.
- Then simply type “node helloworld.js” and hit enter. If you did saved the file with a different name, then use that name. You should see and output of “My name and age is Justice 20” printed on the terminal if everything went successfully. You can check the image below and follow the steps I used to execute the file.
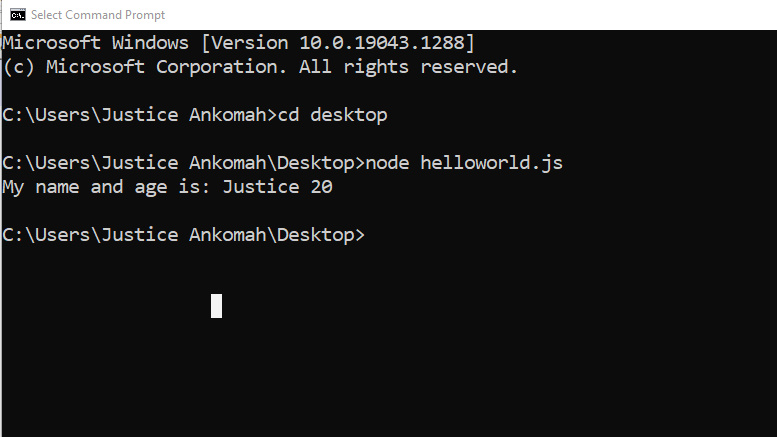
Example 2 – Run Js file in terminal with NodeJs runtime Environment.
Let do another simple example, create a js file and name it “addition.js” and copy and paste the below addition of two numbers function into the file.
// create a function
function addNumbers(num1, num2) {
return num1 + num2;
}
// execute the function
console.log(addNumbers(20,20));
Am very sure you understand what is going on in the script above? It’s just a function that will add any two numbers passed into the function and output the result.
It’s your turn now, follow the above approach I used in the first example to run this file in the terminal. Remember, the file name is “addition.js” and don’t forget to navigate to that path first.
That’s it, you have successfully learned how to run any JavaScript code in the terminal.
How to Run JavaScript File in HTML?
JavaScript is an interpreted language that was developed to help make web pages interactive. It’s not a high-level programming language like JAVA and C++ but it doesn’t mean it’s useless, no you can use JavaScript to develop both front-end and server-side applications with the help of NodeJs.
JavaScript is mainly used in web development especially on the client-side. For example, let say you have a button on your page and you want something to happen when the button is clicked. Well, you can’t use C++ or JAVA for this, it’s the job of JavaScript. If that is clear, let look at how we can run js script with HTML.
There are 2 popular ways for running javascript in HML:
- HTML Inline Javascript code Execution
With this approach, you can decide to put your JavaScript code inside the head or before the closing body tag of your HTML file. For good performance and to ensure that the Dom elements loads before the js it’s recommended to put it in right before the closing body tag.
Example: Create a new .html file and copy and paste below code into it. Notice where the <script></script> tag is placed carefully.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Hello World!</h1>
<!--Put your javacript code here-->
<script>
console.log("inline javascript in html");
</script>
</body>
</html>
Then open the above HTML file in the browser and click to inspect tools. Then navigate to the console tab and you should see “inline JavaScript in HTML” printed.
- Execute External JavaScript File In Html
If you are going to develop a big application that will involve a lot of code, then I recommend that you go with this approach. It’s the best option and it helps you to keep clean and well organized. Here instead of putting all your JS scripts in the same HTML file, let us do something different. The concept is to keep your js code separated from the HTML. So in short, we are going to create an external js file and link it to our HTML.
Example: Create a JavaScript file and name it “myexternal.js” and put the below code into it.
alert("am external script");
Create another file and name it index.html. Copy and paste the below code into it but pay very close attention.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Hello World!</h1>
<!--Link your external javacript file here-->
<script src="myexternal"></script>
</body>
</html>
If you take a good look at the above code, you could see that it has an “src” attribute in the opening “<script>” tag which is pointing to our JavaScript file. It simply means we are making our Script file available to the HTML Dom element so that we can further access them to make our page interactive. That’s all you have to do, you can go ahead and open the file in the browser and you should see a popup alert. I think you have now understood the question well? How to run a JavaScript file?
Check also, JavaScript vs python: which is better?
Including JavaScript – HTML and CSS
Including JavaScript in a webpage or website isn’t that difficult but I want to give you a very quick tip that most people find very confusing. You can use JavaScript to program HTML Dom elements and as well create and remove the existing elements.
But what about CSS? Can you directly link a JS file to it?
The short answer is no, you can use JavaScript to write CSS to style your Dom element by doing something like:
document.getElementById(“p”).style.color = red;
You don’t have to reference any of your JS script or files in your CSS because all of the CSS properties are available to be accessed with JavaScript. It’s very important and you should note it very well in other not to keep confusing yourself. You will understand this clearly when you progress more on learning JS concepts, web development, and developing more JS projects.
Server Side and Client Side JavaScript Execution Difference
The general purpose of JavaScript was to help make web pages interactive. But it developers have worked very hard by coming up with a framework called “Node.Js” which interprets JavaScript on the server. So what exactly is the difference between the two? Well, if JS runs on the browser then it’s a client-side script, but if it runs on the terminal then it’s a server-side script.
If you will recall, you will know that we executed our first script on the command prompt with the help of Node.js. We didn’t link it to any HTML file but we were able to run it successfully why? Its runs on the terminal as a server. But what about the last two examples that run in the browser? Those are client-side JavaScript and we can’t run those as a server-side script.
This gives you an opportunity to decide either you want to focus on front-end, back-end, or full-stack JS development.
How to run js file in browser?
All JavaScript files linked into an HTML file can be run directly in the browser without any compilation needed. Each browser has an engine for interpreting JS scripts. All you need is to make sure your script is linked to your HTML file either inline or externally. Then simply open the file in the browser to see it. Sometimes you might do something wrong and encounter an error.
If that happens you can open the “inspect tool” which is available in almost all browsers including chrome and Mozilla Firefox. By clicking on the console you can see what is wrong and take the necessary steps to fix the bug. The console also gives you the opportunity to write any JavaScript code in it directly. I personally prefer doing that since I could highlight my Dom element and see which one I can make reference to directly for debugging.
Many IDE like visual studio code and Atom also allows you to execute JavaScript code in it directly. Especially VS-Code has a built-in terminal that you can use. It also has popular extensions for previewing Html pages linked with js files directly without using the browser.
Conclusion – Concepts for Running JavaScript Files
You have come a very long way and you have indeed learned a lot in this guide. As I keep saying, I want the best for you so keep practicing and you will master everything. And always remember this whenever you make up your mind to learn something “No one is genius, is all about learning. If someone looks like a genius to you, it simply means such person has learned more stuff than you do.”
You can learn anything in this world if only you really want to. One tip I want to give you in learning JavaScript is that you should try and master the fundamentals of a particular concept before you jump straight to the other. If you don’t put in the time to understand the fundamentals, you might think learning it is hard.
For example, you know they say that if you understand an object then you understand JavaScript right? Why because everything in JS is almost an object. So let say you didn’t put in the time to get a deep understanding of the object. Ask yourself how you will consume JSON data from the server?
Check also, JavaScript vs JQuery difference.
So my little advice is that you should take your time and learn whatever you can. Coding isn’t a competition, the main purpose of coding is for problem-solving. So the key is to figure out some of the problems that exist in your environment and see how you can solve them with your coding skills. And as the top tech saying goes “you earn based on the proportion of the problems you solve.” Any senior developer knows this very well and that’s what pushes them daily to not give up on their skills.
That’s a little tip for you. If you like it or you have any add up to this guide, please let me know in the comment box below.
Now, that you have mastered how to run JavaScript files in the terminal and in HTML. There is one important thing you should know, it’s very beneficial and you will definitely be asked about it in any of your tech interviews. The difference between source code and object code, it really a very important concept you need to understand. Most recruiters ask questions about it to test your fundamentals in programming. Fail it and you will be seen as inexperienced, click on it and take your time to learn all the step-by-step guides I have provided.