The error “Not all code paths return a value” in TypeScript occurs when you do not return a value from a function that needs to return something to the calling code. When the function is executed and the compiler hits the end of the code-block without a seeing a return statement, it will throw the above error indicating that some value needs to be return. Also, if you have multiple conditions, there must be a return value when any of the conditions are met. For example, if you have 10 different conditions in a switch statement it must all return a value including the default condition. In your ‘tsconfig.json’
file, you can set ‘noImplicitReturns’
to ‘false’
or return code paths in the function to fix the error.
When the noImplicitReturns is set to true, typescript will check each code paths in a function to ensure they return a value taking into an account the conditions. That’s that mean when I set it to false the error will be gone totally? Yes, typescript will not notify you of the error not all code paths return a value again but your code will be prone to threat. Because of Linter Rules and typescript configurations, I don’t really recommend disabling the ‘noImplicitReturns’
what you needs is to fix the underlying cause of the error. Below code is an example of how the error occurs…
const getDrink= (val: string) => {
if (val == "orange") {
return "orange juice"
}
else if (val == "apple") {
return "apple drink"
}
else{
// Error: Not all code paths return a value index.ts(4010)
}
}
Above code will throw an error, why? The else block will not return anything when that condition is executed. But if you disable the ‘noImplicitReturns’
you will be able to run the code even though it contains a bug. That’s why I don’t recommend that approach to fix this error, you will be questioned a lot by your team during code review to explain why some conditions don’t return any value.
How to fix Not all code paths return a value error in above typescript code?
const getDrink= (val: string) => {
if (val == "orange") {
return "orange juice"
}
else if (val == "apple") {
return "apple drink"
}
else{
throw new Error('No apple match');
}
}
We have thrown a new error in the ‘else’
block when that condition is fired. The calling code can then catch the error and further up with other operation. You may decide to return null in the ’else’ block depending on your project requirement but I found out that throwing an error is much better. This will fix the error because there’s a return value for all the conditions. You can also set ‘noImplicitReturns’
to false in your ‘tsconfig.json’
file as…
{
"compilerOptions": {
"noImplicitReturns": false,
}
}
Let me show you examples of other reasons why this error occurs…
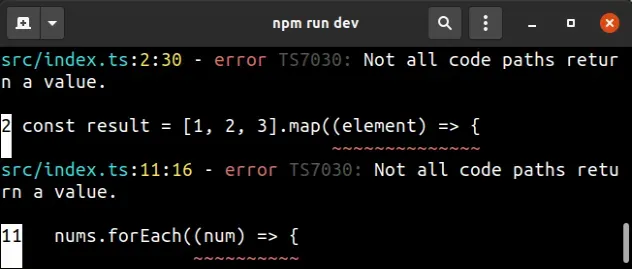
// First example
// ⛔️ Error: Not all code paths return a value.ts(7030)
const result = [1, 2, 3].map((element) => {
if (Math.random() > 0.8) {
return element * 5;
}
// 👉️ Missing return statement here causes an error.
// if the condition returns false, it a big bug
});
// second example
function doCalculation() {
const numbers = [1, 2, 3, 4, 5, 6];
// ⛔️ Error: Not all code paths return a value.ts(7030)
numbers.forEach((num) => {
if (num === 0) {
return num;
}
if (num % 2) {
return num * 2;
}
// 👉️ returning from forEach callback is a mistake
});
return numbers;
}
Conclusion
In conclusion, all that am saying is that the above error occurs when some part of your typescript code does not return a value as required. There are two ways to fix this bug, first is the option I don’t recommend which is to set ‘noImplicitReturns’
to ‘false’ in your ‘tsconfig.json’
file. The second is to make sure to return a value in all your conditions at where it needed.