The “ImportError: cannot import name ‘force_text’ from ‘django.utils.encoding'” error will occur in Django starting from version 4.0 when the ‘force_text’
method is removed and replaced with ‘force_str’
. If the function force_text()
cannot be found in Django.utils.encoding module, it will throw the above error. First, you need to know the purpose of the Django encoding module and why they’re handy when working with text data. If you’re working with text in your Python/Django application it’s obvious that you will surely encode and decode text string to/from byte string and use it in various areas of your program. The encoding module is what you will need because it provides functions or methods for encoding and decoding text Strings to their respective byte code in python.
The easiest approach to solve this error is to upgrade the package that might be the cause of the issue and also correct any import statement that the package depends on.
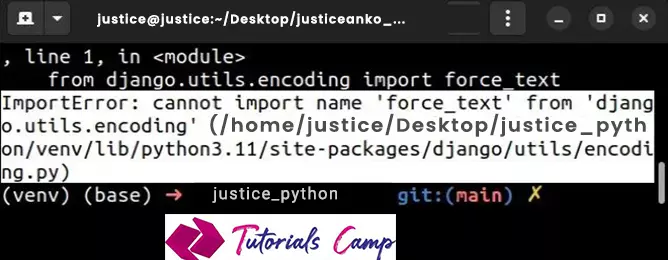
Below is the main text I want you to focus on from the above bug:
ImportError: cannot import name 'force_text' from 'django.utils.encoding' (/home/borislav/Desktop/bobbyhadz_python/venv/lib/python3.11/site-packages/django/utils/encoding.py)
During the release of Django version 4, it was stated that the encoding module ‘django.utils.encoding.force_text’
has been removed. So, we don’t have ‘force_text’
but we rather have ‘force_str’
when importing change from django.utils.encoding import force_text
to from django.utils.encoding import force_str
.
This proves that when you have an outdated package this will be a common reason for the cause of the error, when in your code you try to import ‘force_text’
from ‘django.utils.encoding’.
But the good news is that, when a bug arises, the error message will surely contain information about the package that might be the cause of the error. There’re many packages but the common ones are:
A quick hack is to make sure that you have the latest version of all your packages. Packages can be upgraded by including ‘--upgrade’
option at the end of the ‘pip install’ command when installing any package.
# Command to upgrade graphene-django
pip install graphene-django --upgrade
# Command to upgrade django-elasticsearch-dsl
pip install django-elasticsearch-dsl --upgrade
After upgrading your packages, if any error occurs, you have to change the import statement:
# This old import (Django < v4) will cause an error
from django.utils.encoding import force_text
# Replace the above with this correct import statement (Django v4+)
from django.utils.encoding import force_str
And as I have already told you, if your projects run on Django version 4.0 and above, use force_str and not the deprecated ‘force_text’
(Check and make the necessary changes please). However, if you’re unsure of your project’s Django version you can check it with ‘pip show django’
command.
pip show Django
# or using pip3
pip3 show django
python -m pip show Django
# or using python3
python3 -m pip show Django
Depending on your project setup, operating system, and it environment maybe none of the above solutions will help. If that happens then try adding below code sample at the top of your ‘settings.py’
file.
import django
from django.utils.encoding import force_str
django.utils.encoding.force_text = force_str
From the above code, we first import Django as well as ‘fore_str’
method and then we set ‘force_text’
attribute to ‘force_str’
. By this, if any modules try to access ‘force_text’
they do access ‘force_str’
instead.
There’s one other approach to do this that I don’t recommend, but in case you need:
- First, remove the 3 lines above the file ‘settings.py’
- Then you have to downgrade Django to a version below 4.0 or to a version that you could use the ‘force_text’ method and then change the import statement to the old one
from django.utils.encoding import force_text
#force install Django to the last version below 4.0
pip install "Django<4.0" --force-reinstall
pip3 install "Django<4.0" --force-reinstall
# In case you don't have pip in PATH environment variable then run
python -m pip install "Django<4.0" --force-reinstall
python3 -m pip install "Django<4.0" --force-reinstall
# 👇️ py alias (Windows)
py -m pip install "Django<4.0" --force-reinstall
# This is for those using Jupyter Notebook
!pip install "Django<4.0" --force-reinstall
Try and run the command and if no error occurs then you’re good to go. However, you might encounter an error saying
“ERROR: pip’s dependency resolver does not currently take into account all the packages that are installed.” But don’t be worried because the package will still be installed successfully.
Also, if your project runs on Django version 3, then using the following import statement in your code might help.
from django.utils.encoding import force_text
// Try and print it to see if everything works alright.
print(force_text)
You could also try upgrading all your packages in your environment if only none of the above-suggested solutions help. That means using the deprecated ‘force_text’
function might not be the cause of the error but something else.
Upgrade all packages in your environment to fix importerror: cannot import name force_text from django.utils.encoding
There’re many approaches to upgrading all outdated packages but the most straightforward and simplest way is to use a python script.
import pkg_resources
from subprocess import call
packages = [dist.project_name for dist in pkg_resources.working_set]
call("pip install --upgrade " + ' '.join(packages), shell=True)
Alternatively, you could also store the script in a python file and run a simple command to update all packages. Example. Store the script in ‘main.py
’ and then run the file with the command ‘python main.py’
to upgrade all of the outdated packages.
Depending on the operating system you’re using, that’s either Windows, MacOS, or Linux below are alternative commands to upgrade all outdated packages.
# This is for macOS or Linux users only
pip install -U `pip list --outdated | awk 'NR>2 {print $1}'`
# For Windows users only
for /F "delims= " %i in ('pip list --outdated') do pip install -U %i
If you did use ‘requirements.text’
file, then you can update the packages with the below command.
pip freeze > requirements.txt
Note: when you’re done upgrading Django to the latest version, don’t forget to use the following import statement.
# This is the correct import statement (Django v4+)
from django.utils.encoding import force_str
After, you can go ahead it and use it in your code as shown below:
from django.utils.encoding import force_str
def process_data(input):
the_input_str = force_str(input)
processed_input = the_input_str.upper()
// print it to see the result
print(processed_input)
input = b'Solving importerror: cannot import name 'force_text' from 'django.utils.encoding'’
processed_input = process_data(input)
Output
Solving importerror: cannot import name 'force_text' from 'django.utils.encoding'
The above Django code example illustrates how the process_data()
function/method takes a byte string as input and pass to the force_str()
method to convert it to a text string. Now when the function is called, the force_str(
) decodes the byte string to a text string and returns it.
Conclusion
The main cause of the importerror: cannot import name force_text from django.utils.encoding error is the ‘force_text’
function being removed starting from Django version 4. All you have to do is to replace it with ‘force_str’
and upgrade your packages and correct the import statement and the error will be fixed.
Or simply add the below code to your ‘requirements.txt file and the error will be fixed:
django<=