Master laravel passport API authentication in this two minute tutorial. Securing your API is one of the most essential things every programmer should pay close attention to.
Just as you will not allow anyone to enter into your room except your kids, so it should be when it comes to API development.
Because imagine you have secret details stored in your database. Anyone who will know the URL of your API will surly makes a request to it. But the question is, will you open your door for anyone passing by to enter in?
If no, then you should not expose your API to the general public too. This is because most people cannot be trusted and you may not know the sort of things others will use your API for.
So, how can you secure your laravel API?
Well, there are so many ways you can secure an API. You may choose to use third party libraries or do everything manually yourself. So which is which? Well, it all depends on your choice and how secured you want your API to be.
When it comes to laravel, there are three options:
- Secure it manually yourself by generating and storing random keys for each user of your API. (Remember when I mean users of your API, it means front-end applications like angular, VUE and other mobile apps that will consume your API)
- Use laravel passport 0auth2.0 (third party library)
- JWT (Third party library)
So which one should you use?
Well, as I said it already. It actually depends on how secured you want your API to be and your personal preference.
Use passport 0auth or JWT. And forget about the first option above because random keys generation can be hacked easily. It has happened to me before when I coded a user registration form in complete PHP and used md5 to hash the user’s password.
It’s so easy to reverse keys hashed with md5. So don’t be a victim, don’t manually secure your API with md5 random numbers. Use JWT or Passport.
But I will highly encourage you to use passport because it follows 0auth2.0 concepts. And also all social logins functionalities like Facebook, twitter and Google uses the same. That means when you master laravel passport, you can easily integrate social login functions into your application.
And if you think passport is so hard to understand, then am going to make it easy for you. But before I show you how to use it, let me show you exactly what is it and the whole concept behind it.
What is laravel passport?
Table of Contents
The official definition from the horse own mouth is “Laravel Passport provides a full OAuth2 server implementation for your Laravel application in a matter of minutes. Passport is built on top of the League 0Auth2 server that is maintained by Andy Millington and Simon Hamp.”
It sounds weird right?
All that it means is that, passport is already built on a different server called “League 0Auth2” and you don’t need to create everything from scratch. You just have to install it and bam, you are good to go. Check the below image and you will see that the main ApI and the OAuth2 exist on a different server. Don’t be worried of what is going on in the image, It’s easy and I will show you soon.
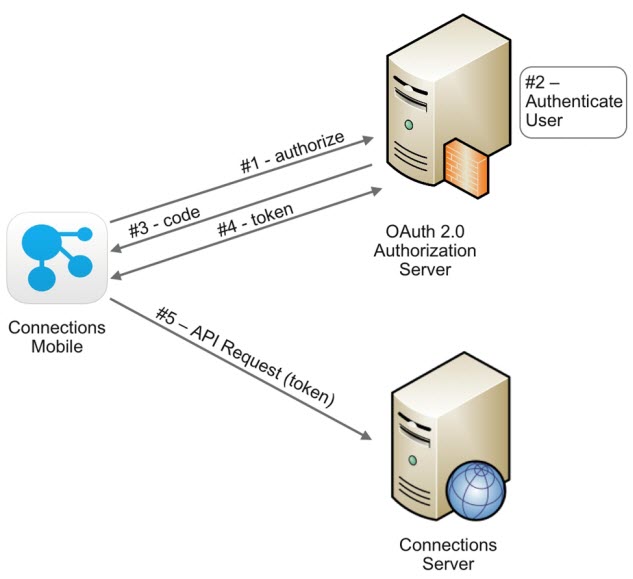
But what is 0auth2? Well, it’s the most preferred method and the most secured way to authenticate an API users before authorizing it to perform any crud action. Now, you are fully confused right? Simply because I just mentioned Authentication and Authorization. Don’t be scared of it, those two words are not Atomic bomb, I have explained it below.
What is ApI Authentication?
Authentication is simply a way of identifying a certain user before you allow him or her to take any action. Here is a real world simple example.
A street guy from nowhere went into a student’s class. All the students including the teacher felt amused, then the teacher asked “Are you part of this class?” No am from the street not a student, he replied.
When the teacher asked if the boy was part of the school, he was actually authenticating his identity to see who he was.
The concept is the same with API authentication. When a user want to fetch an authenticated passport API, the API will ask the user of a token key first. If the user provides the right token key, the API will know that such user is a registered member who is allowed to access the API.
But suppose the user provides wrong or no token key. The API server will authenticate the user and find out that he is not a registered member and such user shouldn’t be authorize of any request.
What is API Authorization?
Whiles authentication is the process of verifying user identity. Authorization is the process of allowing a user to perform a crud operation after it has been authenticated. So remember, without authentication there is no authorization.
Continuing the above real world example to explain authorization.
Will the teacher allow the street guy to study in the class or not?
Well, since the guy was not one of the registered students of the school, the teacher said to him “get out before I call the police, we only teach registered student here” The boy left peacefully.
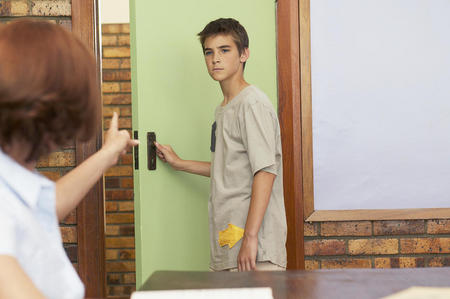
That is what is called authorization and we follow the same approach when developing API’S. If a user identity is not authenticated as a registered user of a particular API, the API will redirect him back to a login page or show a JSON error message like error: “not an authenticated user” without authorizing him to perform any crud operation
How laravel passport works?
Now, let me give you an important concept on the workflow of laravel passport. Let say I have a student’s API that I want to authenticate it with passport. I will first install passport in my app with composer and tell passport with some basic commands that I want to authenticate every incoming API request.
But passport will be like…
“Hey man, if you want me to authenticate every request to your students API, then here is a deal. I will issue a token to all your API users when they register and login. So that with such tokens, I will remember your users and know which request to authorize and which one I should redirect it back. But hey, if you do agree to this deal, then tell all your users to include the token I will give them in every request. If not I will not authenticate such user.”
Pretty simple right? Wrong. You also need to see below analogy to fully understand it. Don’t be worried if you don’t get what is going on in there. I don’t expect you to because am now going to show you how you can work with passport.
How To Use Laravel Passport
Now, that you have gotten a basic idea of passport, it time for us to see how we can actually use it in a real world project. I promise I will make it very, very simple for you to get the whole concept. So that you can use it in whatever way you like in all your project.
But in order to understand it fully and use it in whatever way you want, you need to understand the whole passport concept. That’s one big mistake most programmers repeat it over and over.
They will like to understand the code but not the concept behind. And because of that they end up doing things in one way simply because they don’t actually know the concept behind the code they sometimes write.
But that’s not what I want for you.
Take your time and understand the whole concept behind everything you wish to learn, and you will never go back to relearn it again. Just to give you an example, We all know that the concept of a noun is anything that has a name. Some students will prefer to only memorize the definition and pay no mind to the concept.
Check also: Java vs JavaScript
And because they don’t understand the concept, if being asked some examples of a noun, they may need help from a book. And if you think this is different from learning to code, then think again how many times you have learnt the same thing over and over?
That’s the price of memorizing a code without understand the concept behind.
Sometimes it takes me two good weeks to fully understand the concept behind something new. And it worth it because, once I understands it, it stays forever and I use it in whatever way I like. So…
That’s a little tip for myself.
And you know my name? Am “Justice.”
And you know the whole concept of laravel passport? It’s seen below.
Laravel Passport Concept (They Don’t Want You To Know)
In short, whenever an API is authenticated with passport 0Auth, Any user (front-end web apps, and mobile or desktop apps) who want to make a request to the API will be required to do so by sending his personal token in the headers.
Then what follows is this, Passport will authenticate the user to verify that if such token is correct or not. If the token is correct, Passport will authorize the user to the laravel API server to perform some crud operation.
But if the user sends no token or a wrong token, passport will issue a json error message to the user, alerting it of not authenticated.
That’s the whole concept. The choice of response error and time of issuing a token is granted to every programmer base on his own preference. Others prefer to issue a token to users during registration only, others prefer during login only and others prefer both (one is not bad than the other, it all follows the same concept).
But I personally prefer to send a success response to my API users after registration like, “success: Login to see your access token”
Below image explains the concept I just explained visually. But remember, when the client response is coming back, it does not pass through passport, no. Don’t be worried if you don’t get it, let do an example…
Prerequisites To Create laravel Passport API
We are now going to see how we can create our first ApI. It’s really going to be easier than you think.
But hey, can you access the internet without needing an internet connection and a laptop or smart phone?
If yes, then it’s only in your own world my friend. But…
Am sorry, that’s not how it works in our world of developing laravel API with passport authentication.
We surely need:
- PHP 7+, MySQL, and Apache (if you want to install all of it at once, I recommend you use XAMPP.)
- Composer to install laravel and some passport dependencies
- Laravel 7
- Laravel Passport. Since APIs are generally stateless and do not use sessions to keep track of data, we will use tokens to keep state between requests. Laravel uses the Passport library to implement a full OAuth2 server for authenticating APIs.
- Postman or Insomnia to test our API. From the two, you can choose from any it base on personal preference.
- Any Text editor of your choice, but I will be using visual studio code.
Laravel Passport Oauth2 Example Of API Authentication
Now that you have gotten all the prerequisite set on your system, let the game begin. Develop an API for Bank of America with authentication for $100,000 in 2 minutes.
Can you?
Call me for the money when done, funny. (Don’t mind me, I have not taken my drug today. ha ha-ha, I lough enter mall)
We are going to build a basic API where users will be allowed to see it details after authentication. If others users try to access our API with fake token or no token at all, we will send them an error message to register for a token first.
With this knowledge, you can go ahead and develop any type of API with laravel passport authentication.
Check also:: Illustrator shape builder tool
Creating The API Project
Open your command prompt, navigate to your preferred directory and type the bellow code to create project. Remember, you can call it whatever you like but changing the last word “laravel new Your-preferred-name”
Laravel new laravel-api
Then navigate to the project directory with the below code. Remember to change the last name if you used a different name for your project.
cd laravel-api
Then type “Code” to open the project in visual studio. You should see the project structure like what is in the below image…
Pay no mind to the content on the right. I have not taken my drug today.
Next, laravel has gotten two route service providers. It gives you app the ability to perform normal web http request and API request. But let us disable the web route service and allow only API request.
These two services are provided in “app/providers/RouteServiceProvider.php” boot() function. Simply comment out the web route as I have done it below.
public function boot()
{
$this->configureRateLimiting();
$this->routes(function () {
Route::prefix('api')
->middleware('api')
->namespace($this->namespace)
->group(base_path('routes/api.php'));
// comment bellow web route function out or simply delete it, we won’t be needing it.
/* Route::middleware('web')
->namespace($this->namespace)
->group(base_path('routes/web.php')); */
});
}
Now, your app will only accept API request and that’s what we want.
Don’t be worried, we have not start the server yet. If you want you can go ahead and type php artisan serve on your command prompt and open your browser to http://127.0.0.1:8000/api. You should see your app working (am not doing it to save time).
So let move ahead to..
Laravel passport Installation
I will show you how to build all the routes for the API. But let first install passport…
If you don’t want to run into any issue, please stop your server from running if it’s still running.
Open your command prompt and navigate to your project directory and type bellow code and hit enter. And allow it to install, it usually takes some time, but it depends on your internet speed. Don’t be worried it will not waste all your data (not an atomic bomb)
composer require laravel/passport
Once the installation is complete, a new migration file containing the tables needed to store clients and access tokens will be generated for your application. We now need to run a migration to create the required passport tables. Because mind you, we are going to store the users authenticated tokens.
But before we do, Let configure our database in the .Env file.
Create a database and add the required connection details in the .Env file below. (Am using MYSQL DB with the name “laravel-api”)
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_api
DB_USERNAME=root
DB_PASSWORD=
Now, if we go ahead and migrate our database, our users tables will also be created. But if you don’t like the default column name of the users table, you can adjust it here Database/migrations/2014_10_12_000000_create_users_table.php
public function up(){
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
}
Run the following command to migrate your database to create all the required passport and users tables:
php artisan migrate
When the migration is done, you can go ahead open php/myAdmin and click on your database to see all the tables created.
Next, to create the encryption keys needed to generate secured access tokens, run the below command (don’t close the CLI after this command please):
php artisan passport:install
ON YOUR CLI, IT WILL GENERATE TWO CLIENT ID’S COPY IT AND ADD IT TO THE BOTTOM OF YOUR .ENV
The above command will generate two secrets keys for client_1 and client_2. We need to save in .Env file, and give it to our users to include it in their login body parameters. (That just scared you right? It easy I will show you how)
Copy the two client secrets and open the .Env file. Then paste it as I have done in the end of the file.
CLIENT_1 = hLTaTBunfjzWtEg5pvVFXJhRTdzImyf4BIDu76W8
CLIENT_2 = bDlZOXahYEi9GWB2gn05p1xC6sEKlJxElXZh4806
Then in your “App\Models\User” import the namespace “use Laravel\Passport\HasApiTokens” then add it to “
use Notifiable” property in the User class. Below is what I mean. (If you get error on this step, just restart visual studio code and everything will be fine.)
One of the benefits of this trait is the access to a few helper methods that your model can use to inspect the authenticated user’s token and scopes.
<?php
namespace App\Models;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Laravel\Passport\HasApiTokens; //add this line
class User extends Authenticatable
{
use Notifiable, HasApiTokens; //add this line
protected $fillable = [
'name',
'email',
'password',
];
Next, you should call the Passport::routes()
method within the boot
method of your App\Providers\AuthServiceProvider
. This method will register the routes necessary to issue access tokens and revoke access tokens, clients, and personal access tokens:
public function boot(){
$this->registerPolicies();
Passport::routes(); //add this
}
After calling Passport::routes()above
, Laravel Passport is almost ready to handle all authentication and authorization processes within your application.
Finally, in your application’s config/auth.php
configuration file, you should set the driver
option of the API
authentication guard to passport
. This will instruct your application to use Passport’s TokenGuard
when authenticating incoming API requests:
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'users',
],
'api' => [
'driver' => 'passport', // set this to passport,
'provider' => 'users',
'hash' => false,
],
],
You are done installing passport and is ready to use. Run “php artisan route:list” to see passport available URLS
From the above route list, I have highlighted the URL “oauth/token” which is pointing to this controller “Laravel\Passport\Http\Controllers\AccessTokenController@issueToken” Base on the controller function name, you can easily guess that this is where token will be issued to our users.
Register a user
Now, we surely need to allow our API to be used by others. But not anyone but only those we will grant them the permission.
So let now work on the user’s registration. A users controller is created for you already so open the file “app/http/controllers/userController.php” and create the registration function.
All what is going on in the function is this. Validating users input, hashing the password and storing it in the Users table.
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class userController extends Controller
{
//REGISTER USER
public function register(Request $request){
//validate form input
$this->validate($request, [
"name" => "required|min:3",
"email" => "required|email",
"password" => "required|min:6"
]);
$user = User::create([
"name" => $request->name,
"email" => $request->email,
//hash the password and insert it into db "password" column
"password" => bcrypt($request->password)
]);
return response()->json(["Success" => "Registration Successful"], 200);
}
}
Let provide our users with a URL they can perform the registration. Open route/api.php and just add the below code. I hope it doesn’t need an explanation? If it does, then we are just pointing a URL that will be redirected to the above users registration function we just created.
Remember, you can only access the below URL in your browser with http://127.0.0.1:8000/api/register not http://127.0.0.1:8000/register That’s because we have disabled the web route service. (I hope you do remember?)
//URL to retister a user
Route::post(“/register”, “userController@register”);
Let go ahead and test it by registering a user.
Open postman and make a post request to this URL http://127.0.0.1:8000/api/register with the required body parameters base on the columns on your users table. If you created your users table as mine, then you need only this parameters: name, email and password. Also remember, our users do not need to pass any token in the headers during registration.
Now if you go ahead and hit on send, you should see a success message.
From the above, our user is successfully registered.
He now needs to login to generate a personal access token in laravel passport server.
But the question is, how will the passport server 0Auth2.0 know with api server this user want to authenticate itself to? (Remember there, are other laravel api servers out there like the students one just deployed)
So how will laravel identify your server?
Well, I hope you remember we stored some 2 client secrets in the .env file? Well, those are only the two secret key passport can use to identify any user who want to authenticate itself to our Apl server.
CLIENT_1 = hLTaTBunfjzWtEg5pvVFXJhRTdzImyf4BIDu76W8
CLIENT_2 = bDlZOXahYEi9GWB2gn05p1xC6sEKlJxElXZh4806
So after successful registration of any of our users, we need to show him one of these keys to use it to login to passport server http://127.0.0.1:8000/api/registeroauth/token. He will be required to include it in the body parameters with some other things which I will show you soon.
But now the question is, if we don’t give one of these keys we have stored in the .env file, can they guess it?
No, so why didn’t I show one of it to them after successful registration?
Well, it base on a personal choice, but for security reasons and due to one tip a professional programmer gave me about API access tokens. I usually create a function that will automatically send one of the tokens to the user email. Some users are hackers themselves and others uses software’s that store browser cookies to third party programs. (But do you want one of your client secrets to be used by third parties without knowing their identity?)
And also remember, with one of the above token, multiply users can use it to login to passport server when they get their hand of any of our users details. (Be highly security minded when building API’s)
So after the above user registration, I sometimes simply include this in the response “Note: Your login client id and secrets is sent to your email address. Include it in the body parameters to this url http://127.0.0.1:8000/api/registeroauth/token” But before I show this response, I simple execute a function in the users registration function that will send one of the token to his email address.
But I did not show you this from the beginning because when it come security, everyone follows his own choice and I cannot force you to do things my way.
Now our above registered user needs to login to passport server for an access token that will allow him to perform a crud operation in our API server.
Let see how the login is done, it’s really simple.
laravel passport login
Once a user is registered and being given a client secret key, it really easy for him to register.
Again open your postman and let make a post request to this URL for the login. http://127.0.0.1:8000/oauth/token We need to send the following body parameters: username, password, grant_type, client_id, client_secret and scope. Remember, you need to pass the user email as the his username.
Create Token Laravel Passport
You remember I told you to learn concept not codes right? Yes, because you are about to see a magic bellow when we hit the send button. (While others prefer to create token for users themselves, we have delegated that task to passport server. So don’t scream loudly when you hit the send button and see a token generated for the user)
Go ahead and click on send and you should see a token being generated for the user as shown below.
That’s the token that particular user needs to include it in his http headers whenever he want to access our API.
Laravel Passport 0Auth – Showing Authenticated User Details
Now, let creat a function that will allow the users to see his details.
Open your route.api and add the below code. What is going on is pretty simple, the auth:api middleware will authenticate the user and direct him to the usersdetails function we are about to create in users controllers.
/* middlewaare('auth:api') below is defualt laravel api middlware to allow user perform some operation only when the user is authenticated with token */
Route::middleware('auth:api')->group(function () {
Route::get("/user", "userController@userdetails");
});
If you want to see the middleware class, open your kernel.php file and have a look at it. It should look similar to what is in below code. If not you can adjust it.
protected $routeMiddleware = [
'auth' => \Illuminate\Auth\Middleware\Authenticate::class, // this is the middleware am talking about
'auth.basic' => \Illuminate\Auth\Middleware\AuthenticateWithBasicAuth::class,
'cache.headers' => \Illuminate\Http\Middleware\SetCacheHeaders::class,
'can' => \Illuminate\Auth\Middleware\Authorize::class,
'guest' => \App\Http\Middleware\RedirectIfAuthenticated::class,
'password.confirm' => \Illuminate\Auth\Middleware\RequirePassword::class,
'signed' => \Illuminate\Routing\Middleware\ValidateSignature::class,
'throttle' => \Illuminate\Routing\Middleware\ThrottleRequests::class,
'verified' => \Illuminate\Auth\Middleware\EnsureEmailIsVerified::class,
];
}
We know need to create the function that the above URL will be redirected to. But remember, we only want to show the authenticated user details. So create the below function and add the code in it.
//Get login userdetails
function userdetails(Request $request)
{
return $request->user();
}
If everything is set just as what I have got above, then we are ready to test it out. So open postman and make a Get request to this URL http://127.0.0.1:8000/api/user. Make sure to copy the user token and pass it to the headers as a Bearer authorization.
Go ahead and hit the send button and you should see the users details generated.
So if you are using a vue.js, angular or react.js you can simply include Authorization: Bearer user_token_key in your http headers and make a post request to the same above URL.
If everything works, then congratulations for staying with me to this segment.
Final thought
You can now go ahead and build whatever API project you want and authenticate it in whatever way you like.
Am really sure you have gotten the whole concept behind laravel passport?
If there is anything you don’t understand, you can ask in the input box provided bellow. (I hope the above guide will help you in all your restful-API future projects?)
I just saw my drug, going to take it at the back-end.
Today, while I was at work, my cousin stole my iPad and tested to see if it can survive a 25 foot drop, just so she can be a youtube sensation. My apple ipad is now broken and she has 83 views. I know this is entirely off topic but I had to share it with someone!| Dina Cece Danais
Very hularious
Perfectly written written content , thank you for selective information . Justice your guide is very educative
Am very glade it helps you a lot.
I absolutely love your website.. Pleasant colors & theme.
Did you create this website yourself? Please reply back as I’m wanting to create
my very own site and want to learn where you got this from or what the theme is named.
Thanks!
I created the theme myself and am sorry is not open source to the general public.