Converting string to byte array or bytecode and reverse in java is indeed a good guide that all Java developers need to know. So, in this practical guide, we will create a java program to convert a string to byte-array and you will also find answers to questions like, how many bytes is a string? Is a string a byte array? Is byte [] the same as string?
Converting String to byte[] in Java
Java string class has a metho called getBytes() which returns the number of byte-array of a particular string. It simply converts strings to byte array, so in general this is the method to use if you want to know the byte-array of a string character. The method encodes a string into sequence of bytes of 0s and 1s based on the platform charset and returns a byte array of it that can be stored in a variable for further operations like file handling with file input stream.
You have to take note of two variants when using the getBytes() method to process byte encoding.
getBytes(Character charset) reads a single character and returns it byte
getBytes(String charsetName) read a string and returns a byte array
public class ConvertStringToByte {
public static void main(String[] args) {
String str = "conver me to byte array";
byte[] byteArray = str.getBytes();
// returns the memory address
System.out.println("Array " + byteArray);
// get the byte values
System.out.println("Array as String" + Arrays.toString(byteArray));
}
}
Program Output
Array [B@2c7b84de
Array as String[99, 111, 110, 118, 101, 114, 32, 109, 101, 32, 116, 111, 32, 98, 121, 116, 101, 32, 97, 114, 114, 97, 121]
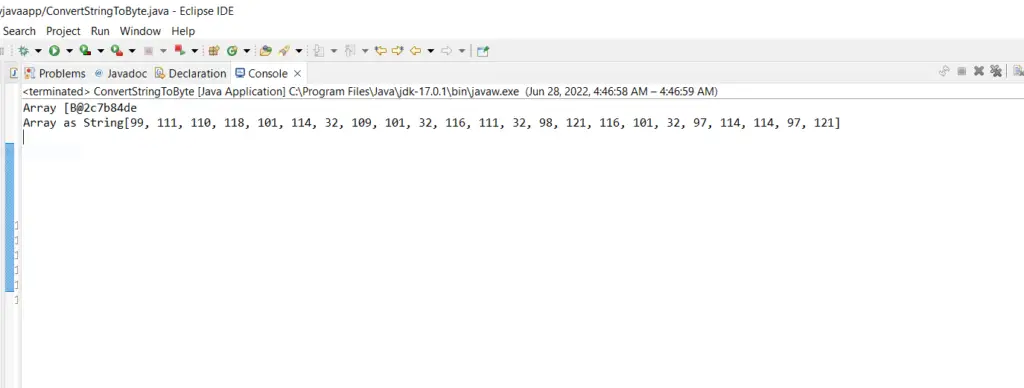
From the above, when the encoded string byte was directly printed, it pointed to the memory address on the operating system. That’s why we used the Arrays.toString() method to print the actual array values.
check also, how to disable button on condition in angular
Conversion of string to byte-array with “UTF-8” encoding
Table of Contents
You can also get the byte-array of a string in Java through UTF-8 encoding, but first let me briefly tells you what is it means. The name ‘’UTF-8” is derived from Unicode or (Universal Coded Character Set) Transformation Format – 8-bit. It actually a set of encoded characters (Unicode) used for electronic communication. Its character encoding format with ASCII looks like on in the picture below…
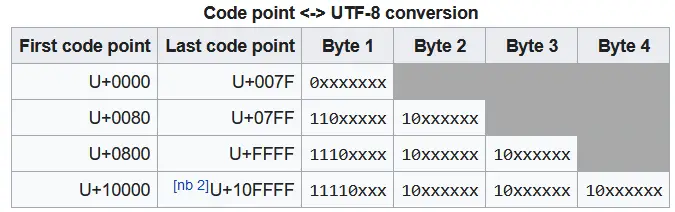
We all know that computer don’t understand English, they process information through binary system of 0s and 1s sequence of characters. An example of byte is “11010101” and encoding is nothing but the process of converting characters in human readable language like English, French and Chinese into binary format that computer understands. Sometimes depending on the strategy being used, encoding can happen in multiple process before a final conversion to byte. Like in the picture below, the characters are first encoded into ASCII-CODE before byte.
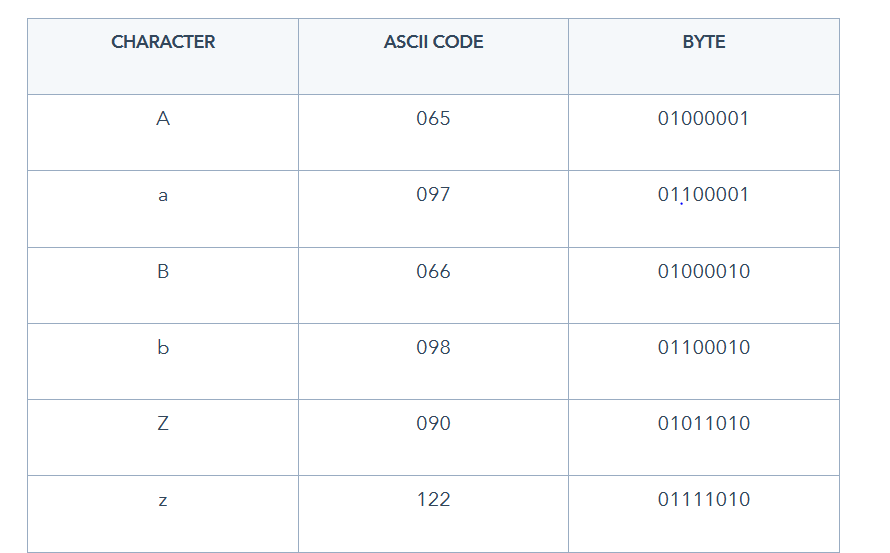
I hope the above guide has given you a real insight of how character encoding works?
Check also, free android app development course for beginners with java programming language.
Let see an example of java program of “UTF-8” and base64 encoding…
public class ConvertStringToByte {
public static void main(String[] args) {
String str = "Java UTF-8 encoding example program";
byte[] b;
try {
b = str.getBytes("UTF-8");
System.out.println(b);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
b = str.getBytes(Charset.forName("UTF-8"));
b = str.getBytes(StandardCharsets.UTF_8);
// old approach, before java 1.7
System.out.println(str.getBytes(Charset.forName("UTF-8")));
// modern approach
byte[] newByte =str.getBytes(StandardCharsets.UTF_8);
String base64encoded = Base64.getEncoder().encodeToString(newByte);
System.out.println(base64encoded);
}
}
Because of UnsupportedEncodingException that can be thrown due to wrong encoding characters, we have surrounded the method str.getBytes(“UTF-8”) that performs the encoding in a try-catch-block to handle the exception when thrown. The program output looks like what’s in the picture below.
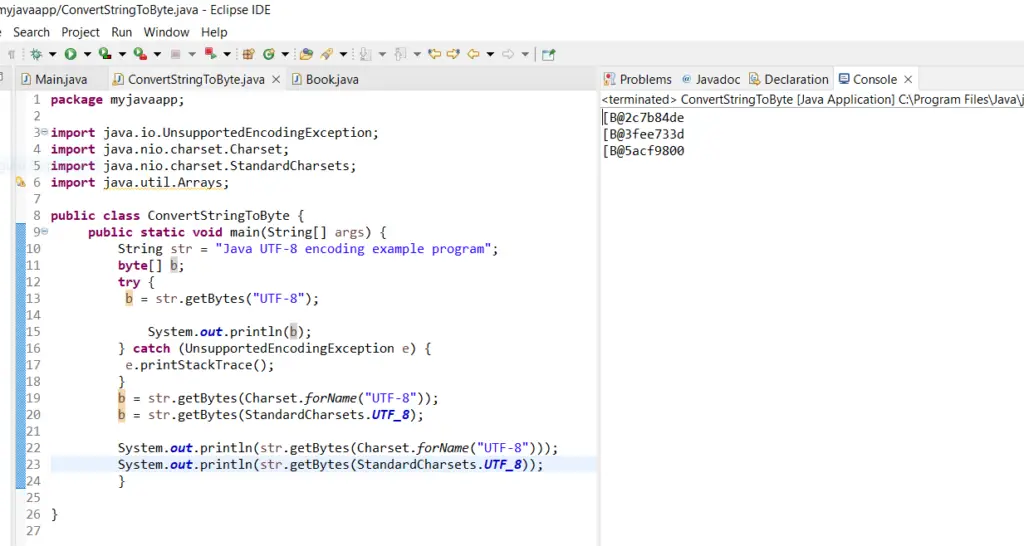
Check also: How to add double quotes around java object and string variables
How to convert/decode byte-array to string in Java
Converting a byte array to string is termed as decoding since the reverse is encoding. What exactly does it mean to decode a byte[] to string? It simply means that if we have converted the sentence “hello world” to a byte array of [0, 123, 34, 001, 345] then it the reverse. This process does requires a charset but there is caution here “In decoding a byte array, you can’t use any charset.” When building a real application, you have to use the charset that encoded the string into byte array for the reverse. This caution is not only in Java programming but almost all the other programming languages.
Java string class has an explicit constructor to convert a byte array to string. The constructor takes in byte[] as an argument and an you can get the decoded string by printing out the object. All you need to do is to create a new string object and pass a byte array into the constructor String(byte[] bytes) using the platform default charset for handling the decoding.
You can also use the same constructor and pass into it a second argument of a different encoding charset. The format looks like this new String(byte[] bytes, Charset charset) but be caution about the hack I told you earlier.
import java.io.UnsupportedEncodingException;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.util.Arrays;
import java.util.Base64;
public class DecodeByteToString {
public static void main(String[] args) {
String str = "Converting string to bytes java programe example.";
// Encode/convert the string to byte[] first
byte[] strByte = str.getBytes();
// Getting the string from a byte array using string constructor
String s = new String(strByte);
System.out.println("String - " + s);
}
}
Program output – Convert String to byte array Java Program (Reverse)
String - Converting string to bytes java program example.
Some one once asked, Which method of String class in Java returns an array of characters as bytes from invoking string? It the same above constructor I have shown you in the example above.
How many bytes is a string?
A single character (char) takes one byte but you can not directly tell how many bytes is a string without computation because its depends on the number or characters that made-up the string. For example these two sentence “hi Java man” and “hi java and python lovers” can not have the same number of bytes due to the char length in each sentence.
Also, the null terminator takes one bytes for a single character which equals N+1. But in UTF-16 also termed as double-byte character the null terminator will be 2N+2. Mostly the encoding and number of characters in the string determines the number of bytes it will take.
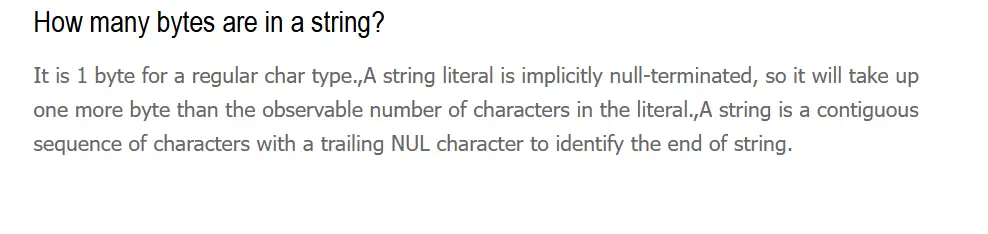
Check also, generating component in subfolder in angular
Convert string to 16 bytes in java
Let say I have a limited storage on my computer that can only stores 16 bytes in byte array. Because of the limited storage capacity, I do understand that If I have a long list of string characters, I can’t use the String.getBytes() for this task.
Imagine a junior dev comes to you for a help on this question, what answer will you give?
Ask yourself, can you really encode string to 16 bytes and decode it back to the original characters? Not possible because by definition, converting arbitrary long complex string to 16 bytes won’t have any impact on the system memory, why, encoding won’t work.
That’s all the guide about “Converting string to byte array Java Program” let me know in the if you have any comment or suggestions.