The python error “TypeError: ‘builtin_function_or_method’ object is not subscriptable” occurs when you call a built in function with square brackets ‘[]’ instead of parentheses ‘()’ Squares brackets are mainly used to perform operations on object that is iterable. For example, if you have a string, list, array or a dictionary, you can use the ‘[]’ to find the index of each particular object in the list or character in a string. That’s the main work of a square bracket, that’s to find the index of an iterable object. You cannot use it to call any of python built-in functions unless that method is iterable.
Let me quickly show you how the TypeError: ‘builtin_function_or_method’ object is not subscriptable error occurs with a code example and how to fix it.
If you have a function that returns a list of strings, it means that function is iterable and you can use the square brackets ‘[]’ on it to find the index of each object being returned. But suppose you want to append an item to a list and you do something like object.append[“hello”]
it will throw an error. The append()
built-in function should be called with a parentheses and not a square bracket because it’s not subscriptable.
ERROR: Exception in Tkinter callback Traceback (most recent call last): File "/tools/python/2.7.2/lib/python2.7/lib-tk/Tkinter.py", line 1410, in call return self.func(*args) File "./file-line.py", line 23, in populate employees.pop[0] TypeError: 'builtin_function_or_method' object is not subscriptable.
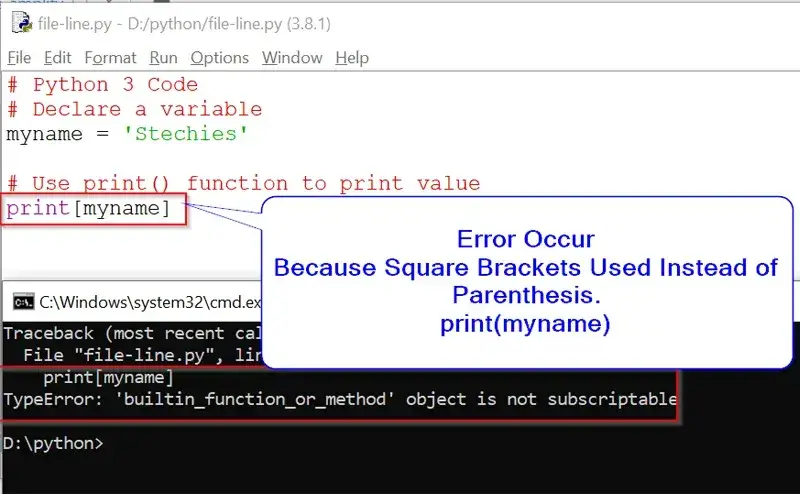
Example
# Declare a list
booksList = ["Head First","Python crash course", "12 python built-in functions"]
# Append an element in the list using append() method
booksList.append[“24 hours python tutorials”]
print(booksList)
Output
booksList.append[[“24 hours python tutorials”]
TypeError: 'builtin_function_or_method' object is not subscriptable
Consider below practical code that uses a square brackets ‘[]’ to access the index of an object in a list.
Programming_languages = ["Java", "Phython", “C++”, “C”]
print(Programming_languages[0])
From the above code, because the variable 'Programming_language'
is subscriptable or iterable, we’re able to access one of its value with an index of 0 surrounded in a square bracket ‘[]’
If we run the above code, it returns 'Java'
which is the first element in the list (note that index of items in a List/Array start from 0). The purpose of the above code is to show you an example of subscriptable or iterable object that you can use square bracket ‘[]’
to retrieve and perform operation on it index. But python built-in functions or methods are not subscriptable because they do not return an iterable object like an Array or a List that you can use ‘[]’
to access it index. Whenever you use a square bracket on an object, the python interpreter will assume that object is itrable and when it notice it’s not, then it will throw an error saying “TypeError: ‘builtin_function_or_method’ object is not subscriptable”
Check also, JavaScript vs python difference
Now, let me show you a simple example code of how the above python error occurs and how to solve it.
Below is a python program that appends an object of students list in a particular city to another list. There’s a condition on how a student should be added to the new school list though (if the student is in that school only). First, let define a list that holds all students objects in the city and an empty school list that a student will be added to.
students = [
{ "name": "John Doe", "in_school": True },
{ "name": "Grace Gate", "in_school": True },
{ "name": "Peter Havard", "in_school": True },
{ "name": "Dereck Java", "in_school": False }
]
school = []
Note from the above code that, because we don’t know yet which student is in the school, we have initialize the ‘school’ list to empty because we want to append each student to the school based on a condition. What we need to do next is to loop through the student list with a for-loop or for-each loop to retrieve each student object and execute the condition on it.
for s in students:
if s["in_school "] == True:
school.append[s]
print(school)
There is an error in the above code but if you’re not careful, you’ll never notice. We’re using the python built-in function append()
to push items to the school list if only a student is in a school otherwise that student object will not be added. Then we used the built-in print()
method to print the list of student in the school.
If we run the program, it will return an error because of this code school.append[s]
above.
Traceback (most recent call last):
File "main.py", line 8, in <module>
school.append[s]
TypeError: 'builtin_function_or_method' object is not subscriptable
How do you fix an object is not Subscriptable in Python? Solution to above TypeError: ‘builtin_function_or_method’ object is not subscriptable error. If you take a good look at the error presented above by python, you can easily spot what causes the bug, the file and the line the interpreter isn’t able to interpret is highlighted.
The error is this code school.append[s]
We’ve called the append method as if it’s an iterable object but it’s not. It just a function and you cannot call it with an array/List syntax using a square bracket. Python built-in Functions are not iterable, so we’ve to call it with a parentheses ‘()’
and not ‘[]’
Let change the code above…
for s in students:
if s["in_school "] == True:
school.append(s)
print(school)
If we run the program, below it’s what it print…
[{'name': John Doe ', 'in_school: True}, {'name': ‘Grace Gate', 'in_school’: True}, {'name': ‘Peter Havard', 'in_school’: True}]
As you can see, the program executed successfully and all that we did was to replace ‘[]’
with ‘()’
What is type builtin_function_or_method?
A built-in function or method is an already defined logics that a programming language like python comes with to be used on it data-types. For example, if you create a variable that has a type of a ‘List’ you can use one of iterable built in methods like append() to add values to the object.
What does it mean in Python when an object is not Subscriptable?
If an object is not subscriptable, it means it’s not indexable so you cannot use the square bracket notation or syntax to call it as you would in a List or an Array. An example of subscriptable object is employees = [“john”, “jame”, “peter”]
and is the same as an iterable object that you can loop through to access the index of each value it holds.
Now, let me give you a quick task. The code below contains a bug, solve it and let me know how it goes in the comment section.
colors = [‘red’, ‘yellow’, ‘green’, ‘blue’, ‘pink’]
# append the new car to the list
colors.append[“white”]
# print the list of new cars
print(colors)
Summary
All that you need to know is that any object that does not return a value that you can loop through does not need to be called using square bracket. Such method might just return a primitive, constant value or void. You need to call python built-in methods like print()
using parentheses and not square bracket.
Thanks for reading and I hope this will help you a lot on your journey of becoming a senior python developer. Now, you can easily solve the above error as an expect whenever you encounter one without any difficulties.