To solve the “error: cannot find module in Node.js or express” you have to first know why this error occurs. Mostly, this error occurs when you’re going through a Node.js tutorial and you reach a point where you need to install some specific module, package or a library. If you don’t properly install it using the npm install
command and you try to import it in any of your project source files with the require()
function, your terminal will throw this below error.
> node app.js
node.js:201
throw e; // process.nextTick error, or 'error' event on first tick
^
Error: Cannot find module 'faye'
at Function._resolveFilename (module.js:334:11)
at Function._load (module.js:279:25)
at Module.require (module.js:357:17)
at require (module.js:368:17)
at Object.<anonymous> (/home/dave/src/server/app.js:2:12)
at Module._compile (module.js:432:26)
at Object..js (module.js:450:10)
at Module.load (module.js:351:31)
at Function._load (module.js:310:12)
at Array.0 (module.js:470:10)
Also, this bug will occur if the library you’re trying to import is incorrectly installed or corrupted.
A quick fix to the error-cannot find module is to try and install all the presented module in your terminal correctly. Navigate to the appropriate directory in your project source folder like app.js or index.js file from your terminal and run npm install package-name
. If that doesn’t work, remove the node_modules
folder that contains the directory for all your app modules. Do the same to the package-lock.json
file and then go ahead to re-install everything again using npm install command.
rm -rf node_modules package-lock.json
npm install
Also, because different operating systems compile special characters differently, make sure your ‘node_module’
folder name does not contain any special characters like hash or space. For example, don’t use path names like ‘myapp/node modules/app.js’
rather use underscore instead of space.
What is Cannot find module? The guide above is just a quick tip and one reason for the occurrence of such error. It’s could be because of below multiple reasons for the “Cannot find module” error in Node.js
- If you’re using typescript instead of JavaScript, it likely that you have forgotten to install type definitions for a particular package in your project.
- Importing a third package that is not installed with
‘npm i thePackage’
will result to an error - Directing or pointing the ‘node’ command to a non-existing file in your project.
- If your IDE, development server glitch or the package version you’re trying to install is outdated. Then it’s reasonable for NodeJS to throw such error.
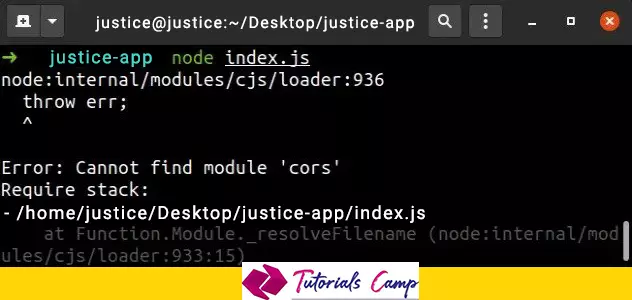
From the above image, you can clearly see the Error: Cannot find module ‘cors’
in the terminal. This indicate that the error is related to the ‘cors’ package not being found in my project. It’s a third-party library we all know. The only way around is to give node what it needs by installing the ‘cors
’ package.
npm install cors
# using TypeScript
npm install --save-dev @types/cors
This will include the package to the dependencies of your project. But if the error is related to a local module, make sure to point the ‘node’
command to the right file by passing in an absolute path the require()
function.
var newPackage = require("./packages/lib.js");
Still if the error persists, try the above quick tip I show you earlier. Delete the ‘node_modules’
together with ‘package-lock.json’
files, install all the packages and restart your IDE and development server because VSCODE sometimes glitches and needs a reboot.
# remove node_modules and package-lock.json
rm -rf node_modules
rm -f package-lock.json
# Also clean npm cache
npm cache clean --force
#then install all packages
npm install
Alternatively, you can install all the packages globally so that you can run it on the command prompt from every directory. To do so use the npm ‘-g’ flag.
npm i -g some-package
#this will globally install the package to local node_modules folder
npm link some-package-name
Sometimes you have to prefix the global installation command with ‘sudo
’ if your attempt fails.
# If permissions error occurs, prefix and run with sudo
sudo npm i -g some-package
npm link some-package
The above npm command creates a global installation source or directory of the package to ‘node_modules/’ folder. And since it globally installed it can be accessed anywhere in your environment.
Also, you can fix the Cannot find module ‘X’ Error in TypeScript project by installing type definitions for all your packages the project depends on with the command ‘npm i -D @types/some-package’
then open your tsconfig.json
file and check if the ‘types’ array contains the ‘node’
string.
{
"compilerOptions": {
"types": [
// make sure this string is present
"node"
]
},
}
After finding the ‘node’
string in the tsconfig.json
file, you can go ahead and run the application and typescript will automatically find type definitions for the ‘cors’
module before compiling the code into Javascript.
Check also:
- Java Vs JavaScript: Which Is Better?
- Fix property assignment expected error in typescript
- Property does not exist on type ‘{}’ in TypeScript
Cannot find Node module in local files
This error is mainly related to the local files you have not a third-party module. It could also be related to a local module you have created yourself. You will experience this error when you pass a wrong local file path to the require()
function in another local file. That’s when you import a file path wrongly. To solve it check your terminal to see a hint of which file the error is related to and change the path in the require()
function.
// import the package first by pointing to the right directory
// then you can go ahead and use it without error
var helperPackage = require("./helpers/lib.js");
// when using ES modules
import helperPackage from "./helpers/lib.mjs";
A similar bug is “error: cannot find module node:events, dotenv or semver” when you’re working with libraries that depends on event.
check also, PHP vs ASP.NET which one to choose for your next web application?
Conclusion
The “Cannot find module” error in Node.js occurs when NODE can not find a specific package your project depends on be it local or third-party library. And the whole concept for solving this error is by making the package available in your project. If it a third-party package, you can install it with the command ‘npm install packageNamge’
however, with local modules, you have to locate the correct path of the module and import it with the require()
function in a file where the module is needed.